The PHP Zip archive extension is used to manage Zip archives and the files they contain. To use this extension in PHP 5, all you need to do is enable the php_zip.dll inside of the php.ini file. In this article, I explain how to manage Zip archive files in PHP using a number of demo PHP applications. Specifically, you’ll learn how to:
- create Zip archives.
- add files and folders inside the archive from a string and from a given path.
- delete and rename files using their indexes and names.
- list the ZipArchive object details (number of files, filenames, comments, statusSys, etc.).
Creating Zip Archives in PHP and Adding Files Inside Them
To create a Zip archive in PHP you can use the predefined constant
ZIPARCHIVE::CREATE
; the archive will be created if it does not exist. (To add files inside an archive you can use the addFile
and addFromString
methods described later.) The first demo application (add_file_from_string.php
) creates (if it doesn’t exist) or opens the archive1.zip Zip archive and then adds a file to a Zip archive using the addFromString()
method, which has the following prototype:bool ZipArchive::addFromString ( string $localname , string $contents ): Add a file to a Zip archive using its contents.
Here is the code for
add_file_from_string.php
:<?php
//Create the ZipArchive object
$zip = new ZipArchive;
//Open/Create if not exist the archive1 zip archive
if ($zip->open("archive1.zip", ZIPARCHIVE::CREATE)!==TRUE) {
exit("cannot open <$filename>"."<br />");
}
//Add a file to a Zip archive using its contents
$zip->addFromString('test1.txt', 'Creating an Zip archive by adding a file to a Zip archive using its contents!');
//Close the active archive
$zip->close();
echo 'The test1.txt file text was successfully added to the archive1.zip';
?>
Here is the output of
add_file_from_string.php
and you can also see it in Figure 1:
The test1.txt file text was successfully added to the archive1.zip
The second demo application (
add_file_directory.php
) also uses the archive1.zip archive to print all the ZipArchive object details (such as status, statusSys, numFiles, filename, comment, or a specified argument, in this case the archive comment and number of files) to add an empty directory named Subdirectory1. This application uses the archive1.zip archive to add a new file as well using the addFile
method. Below are the addFile()
and addEmptyDir()
methods prototypes:bool ZipArchive::addFile ( string $filename [, string $localname ] ) : adds a file to a Zip archive from a given path
bool ZipArchive::addEmptyDir ( string $dirname ) : adds an empty directory in the archive.
Here is the code for
add_file_directory.php
:
<?php
$zip = new ZipArchive();
$zip->open('archive1.zip');
//List all the ZipArchive object details
print_r($zip);
//Adding an empty directory
if($zip->addEmptyDir('Subdirectory1')) {
echo 'Create a new directory'. "<br />";
} else {
echo 'Could not create the directory'. "<br />";
}
//Adding a new text file, the test2.txt
$zip->addFile('test2.txt');
//List the archive1.php comment
echo "Comment: " . $zip->comment . "<br />";
//List the archive1.php number of files
echo "numFile:" . $zip->numFiles . "<br />";
?>
Here is the output of the
add_file_directory.php
listing and you can also see it in Figure 2:
ZipArchive Object ( [status] => 0 [statusSys] => 0 [numFiles] => 2 [filename] => D:Apache GroupApache2htdocsphpZIParchive1.zip [comment] => PHP ZIP ARCHIVE ) Create a new directory
Comment: PHP ZIP ARCHIVE
numFile:4
The next demo application creates the archive2.zip Zip archive, which contains two files: test3.txt and test4.txt. (You will see how to use this application later in the article.) Here is the code for
archive2.php
:
<?php
//Create the ZipArchive object
$zip = new ZipArchive;
//Open/Create if not exist the archive1 zip archive
if ($zip->open("archive2.zip", ZIPARCHIVE::CREATE)!==TRUE) {
exit("cannot open <$filename>n");
}
//Adds a file to a Zip archive from the given path
$zip->addFile('test3.txt');
//Add a file to a Zip archive using its contents
$zip->addFromString('test4.txt', 'Creating an Zip archive by adding a file to a Zip archive using its contents!');
//Close the active archive
$zip->close();
echo 'The test3.txt file text was successfully added to the archive2.zip';
?>
Here is the output of
archive2.php
and you can also see it in Figure 3:
The test3.txt file text was successfully added to the archive2.zip using the addFile method
The test4.txt file text was successfully added to the archive2.zip using the addFromString method.
Extracting a Zip Archive in PHP
The demo application in this section (
extract_archives.php
) shows you how to extract the archive contents into a specified folder, using the extractTo() method:
bool ZipArchive::extractTo ( string $destination [, mixed $entries ] ) : extract the archive contents
. The archive1.zip and the archive2.zip archives will be extracted to the archive folder, as you can see in the Figure 4.Here is the code for
extract_archives.php
:
<?php
$zip1 = new ZipArchive;
$zip2 = new ZipArchive;
//Opens a Zip archive
$extract1 = $zip1->open('archive1.zip');
$extract2 = $zip2->open('archive2.zip');
if (($extract1 === TRUE)&&($extract2 === TRUE)) {
//Extract the archive contents
$zip1->extractTo('archive');
$zip2->extractTo('archive');
//Close a Zip archive
$zip1->close();
$zip2->close();
echo 'The archive1.zip and archive2.zip were extracted in the archive folder!';
} else {
echo 'The extraction of the archive1.zip and archive2.zip failed!';
}
?>
The output of the
extract_archives.php
:
The archive1.zip and archive2.zip were extracted in the archive folder!
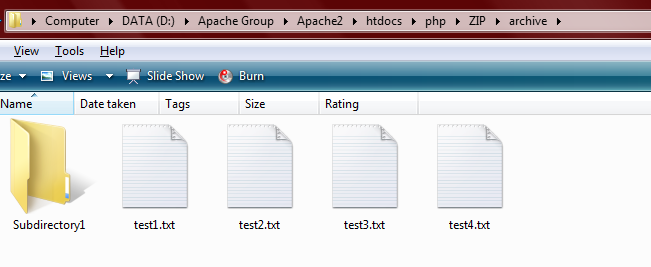
Click here for larger image
Figure 4. The Contents of the Archive Folder, Obtained After archive1 and archive2 Were Extracted
The next demo application (
extract_to_specified_folder.php
) extracts the Zip archive content into a specified folder using the extractTo()
method described above. Here is the code for extract_to_specified_folder.php
:
<?php
//Create the object
$zip = new ZipArchive();
// open archive
if ($zip->open('archive1.zip') !== TRUE) {
die ("Could not open archive");
}
// extract contents to destination directory
$zip->extractTo('../ZIP_extract/');
//Close the archive
$zip->close();
echo "Archive extracted to ZIP_extract folder!";
?>
Here is the output of the
extract_to_specified_folder.php
and you can also see it in Figure 5:
Archive extracted to ZIP_extract folder!
The following demo application (
filelist.php
) extracts from archive2 a file list array containing two files: test3.txt and test4.txt and puts them into the ZIP_TEST folder. Here is the code for filelist.php
:
<?php
//Create object
$zip = new ZipArchive();
//Open archive
if ($zip->open('archive2.zip') !== TRUE) {
die ("Could not open archive");
}
//Extract the selected files into the ZIP_TEST destination directory
$fileList = array('test3.txt','test4.txt');
$zip->extractTo('../ZIP_TEST/', $fileList);
//Close archive
$zip->close();
echo "The test3.txt and test4.txt files from the archive2.zip archive extracted successfully to the specified directory!";
?>
Here is the output of the
filelist.php
listing and you can also see it in Figure 6:
The test3.txt and test4.txt files from the archive2.zip archive extracted successfully to the specified directory!
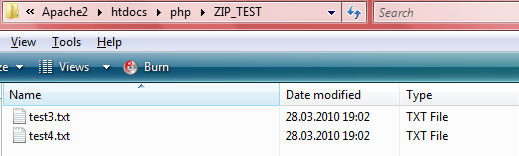
Click here for larger image
Figure 6. The Selected Files Extracted from the archive2 into the ZIP_TEST Destination Directory
Download: php_zip_code.zip