This article was written to provide the novice PHP developer with the tools and skills necessary to obtain dynamic information from one web page and display that data on another web page. What we will be doing is creating our own “weather” script as well as obtaining and displaying the US Department of Homeland Security Threat Level.
We will work through the weather script as a tutorial process, and then we will create and use the Threat Level display as a reference. If you have any questions, feel free to contact me (bpat1434) on the PHPBuilder.com Discussion Forum.
To begin with, let’s show you what we’re going to end up with shall we?
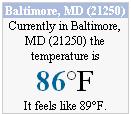
To create the above weather display, you’ll need to visit Weather.com and enter the zipcode you are going to use. For our example, we’ll use 21250 (Baltimore, MD). You will see a page displaying the current temperature, humidity, visibility and other information. For our purposes, we just want the temperature and location. Now let’s look at the code behind the stylish design on weather.com!!
If you right-click and select “View Source” you’ll see the HTML code for that page. If you’re using Firefox, just do a quick find (ctrl + “f”) for the current temperature. The code displayed is as follows:
Weather.com Code:
<TD WIDTH="50%" VALIGN=MIDDLE ALIGN=CENTER STYLE="padding:0px 0px 10px 0px;"> <IMG SRC=http://image.weather.com/web/common/wxicons/52/28.gif WIDTH=52 HEIGHT=52 BORDER=0 ALT=><BR> <B CLASS=obsTextA>Mostly Cloudy</B> </TD> <TD WIDTH="50%" VALIGN=MIDDLE ALIGN=CENTER> <DIV STYLE="padding: 10px 0px 3px 5px;"> <B CLASS=obsTempTextA>83°F</B><BR> <B CLASS=obsTextA>Feels Like<BR> 82°F</B> </DIV> </TD>
That gets us the current temperature as well as what it feels like outside, but we also want the location. Using our code, that’s as easy as typing in the zipcode and searching for it:
Weather.com Code:
<H2 CLASS=”moduleTitleBar”><B>Right Now for</B><BR>Baltimore, MD (21250)<BR>
Okay, so now we know what we’re looking for. How do we actually get that data and put it on our website? We’re going to do it using Regular Expressions. Here are the two patterns we will use:
Regular Expression Patterns
"/<TD WIDTH="50%" VALIGN=MIDDLE ALIGN=CENTER><DIV STYLE="padding: 10px 0px 3px 5px;"> <B CLASS=obsTempTextA>([0-9]*)°F</B><BR> <B CLASS=obsTextA>Feels Like<BR> ([0-9]*)°F</B></DIV></TD>/" "/<TD width="290" ALIGN="left"><H2 CLASS="moduleTitleBar"> <B>Right Now for</B><BR>([a-zA-Z0-9,()s]*)<BR>/"
Now we will explain what these patterns are and what they do. We let the preg_match() function know we are starting a pattern with the forward slash: “/”. We also end the pattern with a forward slash: “/”. The first pattern looks for lines that match our pattern. If it matches, it grabs the unknown values we ask for and returns them. This is the same with the second pattern. The unknown values are characterized by ranges of values. This pattern “([a-zA-Z0-9]*)” searches for any string that is letters or numbers (with no spaces) repeated any number of times. Each time we designate one area as a match, we return another unknown value. So our first pattern returns two, and our second pattern returns one. Each unknown value is characterized by the opening and closing parenthesis “()”.
I’m sure you’ve noticed this weird pattern by now: “([a-zA-Z0-9,()s]*)”. What that looks for is any letter (case insensitive), number, parentheses, or non-breaking space in any order until it reaches the “<BR>” tag. The two backslashes (“”) escape the pattern so that the parentheses () will be interpreted literally.
If that was all really confusing, don’t worry about it. This isn’t a RegEx tutorial. All you need to know about it is that the first pattern will grab the two numerical temperatures, and the second will grab the “city, state (zipcode)” values. So now that we have our patterns, how about we work on getting our script together?
The way we read the page with the weather information on it is by opening a pipe to the site and reading what it gives us back. Using fopen() and file_get_contents() we can get the entire HTML code of the page we want. Here’s how the opening and closing of the pipe should be done:
fopen() Code:
<?php $url = "http://www.weather.com/weather/local/21250"; $handle = fopen($url, "r"); $contents = file_get_contents($url); fclose($handle); ?>
Okay, so the above code will open the web page http://www.weather.com/weather/local/21250 and read its HTML contents into a variable aptly named “contents”. Now that we’ve got our code, let’s see if we can find our temperature!!
PHP Code:
<?php
$url = "http://www.weather.com/weather/local/21250";
$handle = fopen($url, "r");
$contents = file_get_contents($url);
fclose($handle);
$temp_pattern = "/<TD WIDTH="50%" VALIGN=MIDDLE ALIGN=CENTER>
<DIV STYLE="padding: 10px 0px 3px 5px;">
<B CLASS=obsTempTextA>([0-9]*)°F</B>
<BR><B CLASS=obsTextA>Feels Like<BR> ([0-9]*)°F</B>
</DIV></TD>/";
$loc_pattern = "/<TD width="290" ALIGN="left"><H2 CLASS="moduleTitleBar">
<B>Right Now for</B><BR>
([a-zA-Z0-9,()s]*)<BR>/";
// preg_match(pattern, string, returned array)
preg_match($temp_pattern, $contents, $temps);
preg_match($loc_pattern, $contents, $location);
echo 'Currently in '.$location[1].':<br>
the temperature is <b>'.$temps[1].'</b>°F.<br>
It feels like <i>'.$temps[2].'</i>°F.';
?>
And with that, you can make your own weather script for your site!! So now you know the basics of retrieving remote data and customizing the output. In the next page, I’ll show you a more concise example of what you can do to output the data in XML.