Using the Google Books service, you can search and navigate material that previously was available only in print form much in the same way you use Google’s search engine to comb the world’s web sites. Like many of Google’s services, Google Books has an API, which allows developers to create their own applications backed by the massive Google Books database. The Google Books API allows you to search the database, interact with the service’s social features such as retrieve and submit user reviews, view and manage users’ book collections, and even embed the book preview interface into your web site.
For PHP developers, the Zend Framework provides access to the Google Books API via the Zend_Gdata component, which is capable of interacting with most — if not all — of Google’s various APIs. Using this component you can easily incorporate book-related data into your Zend Framework-powered applications, and even create compelling new applications based around this vast literary trove.
Regardless of how you feel about Google’s controversial move to assemble and display part or all of the world’s books via Google Books, you have to admit it’s a pretty interesting and ambitious project. In this article, I explain how to search Google’s books database with the Zend Framework’s Zend_Gdata component.
Introducing the Zend_Gdata Component
The Zend_Gdata component is a native part of the Zend Framework, so you don’t need special configuration to begin using it. All you need to do is invoke the
Zend_Gdata_Books
class and then use its methods. In this section I’ll show you how to take advantage of this class to interact with Google Books in a variety of ways.Searching Books by Keyword
Perhaps the most fundamental task when using the Zend_Gdata component is searching the Google Books database for books containing a particular keyword or phrase. For instance, suppose you wanted to find all books mentioning the term “wind power.” The following snippet will search the database for this term, displaying a list of titles that meet this condition:
$keywords = urlencode("intitle:wind power");
$books = new Zend_Gdata_Books();
$query = $books->newVolumeQuery();
$query->setQuery($keywords);
$feed = $books->getVolumeFeed($query);
foreach ($feed as $entry) {
$link = $entry->getLink();
echo "<div style='overflow: auto; width: 100%; padding: 5px;'>
<a href='{$link[1]->getHref()}'>{$entry->getTitle()}</a><br />";
echo "<img src='{$link[0]->getHref()}' style='float:left;'/></div>";
}
Executing this code produces a list of 10 titles, complete with a link pointing to the book’s entry in Google Books and a thumbnail of the book cover. A sampling of the output is presented in Figure 1.
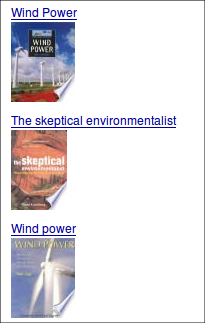
Figure 1. Searching Google Books by Keyword
By default, a search returns 10 results. You can adjust the number of retrieved books using the
setMaxResults()
method. Because many queries will return quite a few entries, you can use the setMaxResults()
method in conjunction with the setStartIndex()
method to create paged output.Also, notice how PHP’s
urlencode()
function was used to first encode the keywords. Neglecting to do this will produce an error, so be sure to do it before executing the search.Refining Your Search
By default, the
getVolumeFeed()
method will return any book in which the keyword is found in the title, author name(s), book text, or other metadata. This could naturally produce quite a few false positives, because many books could contain little more than a passing reference to the provided keywords, rather than anything of substance on the topic. You can refine your search to target specific parts of the book, such as the title, by accompanying the keywords with filters. For instance, to search only the titles of books for a specific keyword or phrase, you would preface the keyword with intitle:
, like this:
$keywords = urlencode("intitle:wind power");
$books = new Zend_Gdata_Books();
$query = $books->newVolumeQuery();
$query->setQuery($keywords);
Several other filters are available, although this particular feature is currently poorly documented. The easiest way to learn more about these filters is by heading over to the Google Books Advanced Search feature and searching the database using one of the preset filters. When the search is performed, you’ll also be able to see which filter has been used, as it will be specified in the search box at the top of the page. For instance, you can search by ISBN using the
isbn:
keyword.
$keywords = urlencode("isbn:0615303889");
$books = new Zend_Gdata_Books();
$query = $books->newVolumeQuery();
$query->setQuery($keywords);