Adding Interactive Row Highlighting
Alright, this is really starting to shape up nicely! I’d like to add one last feature, a bit of interactivity which will bring specific attention to each row as the user mouses over. Believe it or not this is most easily accomplished using CSS’
hover
selector. Add the following to your CSS definitions:
#games tr:hover {
background-color: #A4E666;
}
The
hover
selector works in conjunction with all modern browsers. You’ll also want to comment out or remove the altRowAttributes()
method call. With the CSS in place, any row mouseovers will cause the row to turn green, as demonstrated in Figure 5.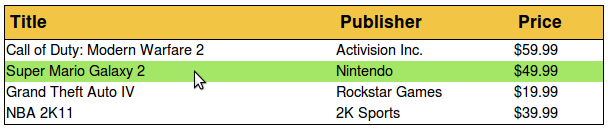
Figure 5. Adding a Bit of Interactivity
Sorting Rows Interactively
While you can make an educated guess regarding how the user would like to sort the table, it’s always ideal to give the user the power to override this default. Add interactive sorting using the Tablesorter jQuery plugin. Once configured, the user can click on any table header to resort the contents. Of course, to integrate this feature you’ll need to reference both jQuery and the Tablesorter plugin in your document. Additionally, you’ll need to reformat the table a bit to use both the
THEAD
and TBODY
tags. I’ve provided all of the code used to integrate this interactive feature in the following listing, highlighting key lines:
<html>
<head>
<script type="text/javascript"
src="http://www.google.com/jsapi"></script>
<script type="text/javascript">
google.load("jquery", "1.5");
</script>
<script type="text/javascript" src="jquery.tablesorter.min.js"></script>
<script type="text/javascript">
google.setOnLoadCallback(function() {
$("#games").tablesorter();
});
</script>
</head>
<body>
<?php
require_once "HTML/Table.php";
$games = array(
array("Call of Duty: Modern Warfare 2", "Activision Inc.", "$59.99"),
array("Super Mario Galaxy 2", "Nintendo", "$49.99"),
array("Grand Theft Auto IV", "Rockstar Games", "$19.99"),
array("NBA 2K11", "2K Sports", "$39.99")
);
$table = new HTML_Table(array("id" => "games",
"class" => "tablesorter"), 0, TRUE);
$head =& $table->getHeader();
$head->setHeaderContents(0, 0, 'Title');
$head->setHeaderContents(0, 1, 'Publisher');
$head->setHeaderContents(0, 2, 'Price');
$body =& $table->getBody();
for ($rows = 0; $rows < count($games); $rows++)
{
for($cols = 0; $cols < count($games[0]); $cols++)
{
$body->setCellContents($rows, $cols, $games[$rows][$cols]);
}
}
echo $table->toHtml();
?>
</body>
</html>
Conclusion
Using powerful tools such as HTML_Table, CSS and jQuery, it really is incredible what’s possible with just a few lines of code. What tools do you use to format your tables? Tell us about them in the comments!
About the Author
Jason Gilmore is founder of the publishing, training, and consulting firm WJGilmore.com. He is the author of several popular books “Easy PHP Websites with the Zend Framework”, “Easy PayPal with PHP”, and “Beginning PHP and MySQL, Fourth Edition”. Follow him on Twitter at @wjgilmore.