Before we can start drawing the graph, let’s start with the basics and look at how PHP creates an image. The first thing that we need to do is tell the browser that it’s getting an image, and what kind of image it’s getting:
<?php
Header
( "Content-type: image/gif");
?>
Now that the browser knows it’s getting a GIF image we can start creating the image. First up we have to create a blank canvas to start drawing on. The
ImageCreate
function does this for us. ImageCreate
will return an identifier to the image and it we need to tell the function is how large to make the canvas in pixels, x (width) by y (height).
<?php
$image
= imagecreate(200,200);
?>
Now we’ve got a 200 pixels by 200 pixels blank canvas to start working on. The next step is to create some colors that are going to be used in the image. For this we have to use the
I’ll allocate a color identifier called
ImageColorAllocate
function, and it needs to know the identifier of the image that the color is for, as well as the RGB value of the color. ImageColorAllocate
will return an identifier to the color that we just created. We will use the color identifier later when we start drawing on the canvas. The way that ImageColorAllocate
works is that we have to allocate a color for each image that we are working on – so if we were creating 3 GIF’s and wanted red in each of them, we would have to allocate the color red 3 times – once for each GIF.I’ll allocate a color identifier called
$maroon
, and give the red value 100, green 0 and blue 0. While I’m at it I’ll create white as well.
<?php
$maroon
= ImageColorAllocate($image,100,0,0);
$white = ImageColorAllocate($image,255,255,255);
?>
Now that we’ve got our color, we can draw something with it. The first thing to do is paint our canvas white. The function
ImageFilledRectangle
will draw a rectangle on our canvas and fill it with the color we specify.
<?php
ImageFilledRectangle
($image,0,0,200,200,$white);
?>
The first thing to tell
ImageFilledRectangle
, as with all Image functions, is which image we are working with, so we pass it the $image
identifier. It then needs to know the x and y co-ordinates to start the rectangle at (0,0 – the upper left hand corner) and the co-ordinates to end the rectangle at (200,200 – the bottom right hand corner of the canvas). The last thing to tell it is the identifier of the color to draw the rectangle in, in this case $white
. Now we can start drawing on our nice white background.
<?php
ImageRectangle
($image,10,10,190,190,$maroon);
ImageFilledRectangle($image,50,50,150,150,$maroon);
?>
ImageRectangle works in exactly the same way as
ImageFilledRectangle
, except that it doesn’t fill the rectangle with color. Once we are done drawing, we can output the image –
<?php
ImageGIF
($image);
?>
And then destroy the image that we are storing in memory:
<?php
ImageDestroy
($image);
?>
What this gives us is:
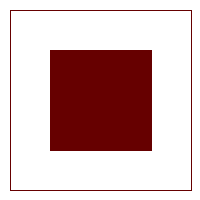
which is not quite a graph, yet.