Whether you’re trying to develop web applications with a truly global appeal, or you’re one of the roughly 300 million United States residents mired in a maddening cacophony of conflicting measurement standards, chances are you’ll need to regularly convert data from one standard to another. Of course, performing even relatively simple conversion calculations such as from pounds to kilograms from memory can be fairly difficult, let alone calculating more esoteric conversions such as from square meters to acres. To automate this conversion process, I used the Zend Framework to create a calculator capable of easily migrating data among the most commonly used formats. In this tutorial, I’ll show you how you can use the Zend Framework’s Zend_Measure component to create your own measurement-conversion calculator.
Introducing the Zend_Measure Component
True to the Zend Framework team’s stated goal of “focusing on the most commonly needed functionality,” they have taken great pains to provide readily accessible solutions for carrying out commonplace tasks that developers around the globe face. The Zend_Measure component implementation is a prime example of this pragmatic approach.
Capable of converting between an enormous number of measurement formats, Zend_Measure is a useful tool for carrying out not only typical conversions such as between miles and kilometers, but also between a wide variety of scientific formats such as torque, frequency, force, illumination, current, and density. For the purpose of demonstration, I’ll stick to some of the more common measurement units, but I invite you to investigate the other supported measurement types on the Zend_Measure documentation page, or perhaps better, browse the Zend_Measure source code directly.
To use the Zend_Measure component, start by defining the originating unit type and value, and then call the
convertTo()
method to convert the value to the desired destination unit type. Consider a simple example that converts between centimeters and inches:$locale = new Zend_Locale('en');
$unit = new Zend_Measure_Length(36, Zend_Measure_Length::CENTIMETER, $locale);
echo "CONVERT: ".$unit->convertTo(Zend_Measure_Length::INCH);
Executing this example will produce the following output:
CONVERT: 14.0 in
Therefore, to convert a value from one standard to another, you statically call the respective class constants, in this case
(Zend_Measure_Length, Zend_Measure_Volume, Zend_Measure_Area, etc.) contains a well-organized list of class constants at the top of the file. In fact, understanding precisely what these constants are will play an important role later in this tutorial when we use reflection to dynamically retrieve the class constant values, depending upon the desired conversion task.
Zend_Measure_Length::CENTIMETER
and Zend_Measure_Length::INCH
. Again, the easiest way to determine which standards are available is by examining the source code. Each Zend_Measure class(Zend_Measure_Length, Zend_Measure_Volume, Zend_Measure_Area, etc.) contains a well-organized list of class constants at the top of the file. In fact, understanding precisely what these constants are will play an important role later in this tutorial when we use reflection to dynamically retrieve the class constant values, depending upon the desired conversion task.
To drive home the syntax pattern, consider another example. This time it converts between acres and square meters:
$locale = new Zend_Locale('en');
$unit = new Zend_Measure_Area(1, Zend_Measure_Area::ACRE, $locale);
echo "CONVERT: ".$unit->convertTo(Zend_Measure_Area::SQUARE_METER);
Executing this example will tell you that 1 acre is equivalent to 4,047 m2.
Creating a Simple Conversion Calculator
Now that you understand how the conversion process works, let’s build a simple calculator using the Zend Framework. Incidentally, you can experiment with the conversion calculator by navigating to converter.wjgilmore.com. I’ll start with the conversion form, which for reasons of convenience I’ve created within the
Index
controller’s index
method view:<h3>Cooking Conversion Calculator</h3>
<?php if (isset($this->convertedValue)) { ?>
<p><b>Conversion: <?= $this->convertedValue; ?></b></p>
<?php } ?>
<form action="/" method="post">
<p>
From:<br />
<input type="text" name="source_val" value="" size="3" />
<select name="source_type">
<option value="CUP_US">Cups</option>
<option value="GALLON_US">Gallons</option>
<option value="OUNCE_US">Ounces</option>
<option value="PINT_US">Pints</option>
<option value="QUART_US">Quarts</option>
<option value="TABLESPOON_US">Tablespoons</option>
<option value="TEASPOON_US">Teaspoons</option>
</select>
</p>
<p>
To:<br />
<select name="destination_type">
<option value="CUP_US">Cups</option>
<option value="GALLON_US">Gallons</option>
<option value="OUNCE_US">Ounces</option>
<option value="PINT_US">Pints</option>
<option value="QUART_US">Quarts</option>
<option value="TABLESPOON_US">Tablespoons</option>
<option value="TEASPOON_US">Teaspoons</option>
</select>
</p>
<p>
<input type="submit" name="submit" value="Convert!" />
</p>
</form>
Figure 1 presents the conversion form as it is rendered within the browser. I’ve purposefully kept the design layout simple in order to ensure proper rendering within mobile browsers, a destination where I’ve found such a calculator to be quite useful.
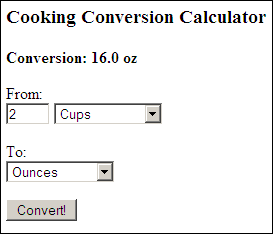
Figure 1. A Conversion Calculator for Cooking Measurements