Logging into the Twitter Account
When building browser-based Twitter clients, the OAuth authentication procedure is a two-step process that requires the user to visit the Twitter website at least once in order to confirm that he would like to provide the application with access to his account details (see Figure 1).
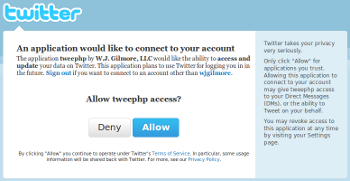
Figure 1. Confirming Access to My Tweephp Application
The code used to carry out this authentication process is undeniably long, made even more so by the fact that I didn’t want to potentially confuse anybody by abstracting away some of the details. I suggest taking a few moments to review the following code, keeping in mind that the OAuth authentication process is accomplished in two steps. The first step (defined by the first part of the
if
conditional) sends the user to Twitter to confirm his wish to grant the application access to his account. The second step (defined in the second part of the if
conditional) retrieves the OAuth access token following successful authentication, allowing the application to begin interacting with the user’s account.
<?php
session_start();
require_once 'Services/Twitter.php';
require_once 'HTTP/OAuth/Consumer.php';
define('CONSUMER_KEY', 'SECRET');
define('CONSUMER_SECRET', 'SUPER_SECRET');
try {
$twitter = new Services_Twitter();
// If the user session isn't active
if (! isset($_SESSION['token']))
{
$oauth = new HTTP_OAuth_Consumer(CONSUMER_KEY, CONSUMER_SECRET);
$oauth->getRequestToken('http://twitter.com/oauth/request_token',
'YOUR_RETURN_URL');
$_SESSION['token'] = $oauth->getToken();
$_SESSION['token_secret'] = $oauth->getTokenSecret();
$url = $oauth->getAuthorizeUrl('http://twitter.com/oauth/authorize');
header('Location: '.$url);
// Authentication successful, retrieve the access token
} else {
$oauth = new HTTP_OAuth_Consumer(CONSUMER_KEY, CONSUMER_SECRET,
$_SESSION['token'], $_SESSION['token_secret']);
if (isset($_GET['oauth_identifier'])) {
$oauth->getAccessToken('http://twitter.com/oauth/access_token',
$_GET['oauth_verifier']);
$_SESSION['token'] = $oauth->getToken();
$_SESSION['token_secret'] = $oauth->getTokenSecret();
}
$twitter->setOAuth($oauth);
}
} catch (Services_Twitter_Exception $e) {
echo $e->getMessage();
}
?>
Keep in mind that the user has to travel to Twitter only once, as not only will a session be active following successful authentication, but Twitter also will know which applications the user has granted access to.
Displaying a Twitter User’s Recent Messages
When authenticated, the application is free to retrieve messages, submit updates, and carry out other tasks on the user’s behalf. For instance, the following snippet will output a list of all of the user’s recent updates:
$response = $twitter->statuses->user_timeline();
foreach($response AS $item)
{
printf("<p>On %s %s tweeted:<br />%s</p>",
$item->created_at, $item->user->screen_name, $item->text);
}
Submitting Updates
To submit an update, just use the
update()
method as demonstrated here:
$msg = $twitter->statuses->update("Browser-based Twitter clients are fun!");
For a complete breakdown of available API commands, see the Twitter API documentation.
About the Author
Jason Gilmore is the founder of the publishing and consulting firm WJGilmore.com. He also is the author of several popular books, including “Easy PHP Websites with the Zend Framework”, “Easy PayPal with PHP”, and “Beginning PHP and MySQL, Fourth Edition”. Follow him on Twitter at @wjgilmore.