Professional with permission from Apress.
set of functionality for creating actions on the fly, if you are not making use
of its ability to connect to the server, you are really just using basic
JavaScript. Not that there is anything truly wrong with that, but the real power
lies in joining the client-side functionality of JavaScript with the server-side
processing of the PHP language using the concept of Ajax.
can be used together to design some basic tools that are quite new to Internet
applications but have been accessible to desktop applications for ages. The
ability to make a call to the server without a page refresh is one that is quite
powerful, if harnessed correctly. With the help of the powerful PHP server-side
language, you can create some handy little applica-tions that can be easily
integrated into any web project.
languages (ASP, ASP.NET, ColdFusion, etc.), why have I chosen to devote this
book to the PHP language, as any of them can function decently with Ajax
technologies? Well, the truth is that while any of the afore-mentioned languages
will perform admirably with Ajax, PHP has similarities with the JavaScript
language used to control Ajax??in functionality, code layout, and ideology.
While code written in PHP is always hidden from the web user, there is a massive
community of developers who prefer to share and share alike when it comes to
their code. You need only scour the web to find an abundance of examples,
ranging from the most basic to the most in-depth. When comparing PHP??s online
community against a coding language such as ASP.NET, it is not difficult to see
the differences.
fact that it does not remain hidden. Because it is a client-side technology, it
is always possible to view the code that has been written in JavaScript. Perhaps
due to the way JavaScript is handled in this manner, JavaScript has always had a
very open community as well. By combining the communities of JavaScript and PHP,
you can likely always find the exam-ples you want simply by querying the
community.
comes down to mere func-tionality. PHP is a very robust, object-oriented
language. JavaScript is a rather robust language in itself; it is sculptured
after the object-oriented model as well. Therefore, when you combine two
languages, aged to maturity, you come away with the best of both worlds, and you
are truly ready to begin to merge them for fantastic results.
sides to a web page??s proverbial coin. There is the client-side communication
aspect??that is, the functionality happen-ing right then and there on the
client??s browser; and the server-side processing??the more intricate levels of
scripting, which include database interaction, complex formulas, conditional
statements, and much, much more.
language to handle the client-side interaction and merging it seamlessly with
the PHP processing lan-guage for all your server-side manipulation. By combining
the two, the sky is truly the limit. Anything that can be imagined can come to
fruition if enough creativity and hard work is put into it.
and involved examples, I will begin by showing some basic examples of common web
mini-applications that work well with the Ajax ideology. These are examples you
are likely to see already in place in a variety of web applications, and they
are a very good basis for showing what can be accomplished using the Ajax
functionality.
chap-ter will attempt to guide you as to what makes these pieces of
functionality so well-suited to the Ajax concept. Not every application of Ajax
is necessarily a good idea, so it is important to note why these examples work
well with the Ajax concept, and how they make the user??s web-browsing experience
better. What would the same application look like if the page had to refresh?
Would the same functionality have even been possible without Ajax, and how much
work does it save us (if any)?
hiding content away and exposing it based on link clicks (or hovers, or button
presses). This sort of functionality allows you to create access to a large
amount of content without cluttering the screen. By hiding con-tent within
expandable and retractable menu links, you can add a lot of information in a
small amount of space.
calendar based upon link clicks. By using Ajax to hide and show information, and
PHP to dynami-cally generate a calendar based upon the current month, you create
a well-hidden calendar that can be added to any application with relative ease
and very little web site real estate.
your calen-dar. The following code will create your very basic web page:
<!-- sample3_1.html -->
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"➥"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <title>Sample 3_1</title> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1" /> <script type="text/javascript" src="functions.js"></script> <link rel="stylesheet" type="text/css" href="style.css" /> </head> <body>
<div id="createtask" class="formclass"></div>
<div id="autocompletediv" class="autocomp"></div>
<div id="taskbox" class="taskboxclass"></div>
<p><a href="javascript://" onclick="showHideCalendar()">➥<img id="opencloseimg" src="images/plus.gif" alt="" title="" ➥ style="border: none; width: 9px; height: 9px;" /></a>➥ <a href="javascript://" onclick="showHideCalendar()">My Calendar</a></p>
<div id="calendar" style="width: 105px; text-align: left;"></div> </body> </html>
//functions.js
//Create a boolean variable to check for a valid IE instance.
var xmlhttp = false;//Check if we are using IE.
try { //If the javascript version is greater than 5. xmlhttp = new ActiveXObject("Msxml2.XMLHTTP");
} catch (e) { //If not, then use the older active x object. try {
//If we are using IE.
xmlhttp = new ActiveXObject("Microsoft.XMLHTTP");} catch (E) {
//Else we must be using a non-IE browser.
xmlhttp = false;} }
//If we are using a non-IE browser, create a JavaScript instance of the object. if (!xmlhttp && typeof XMLHttpRequest != 'undefined') { xmlhttp = new XMLHttpRequest(); }
//A variable used to distinguish whether to open or close the calendar. var showCalendar = true;
function showHideCalendar() {
//The location we are loading the page into.
var objID = "calendar";//Change the current image of the minus or plus.
if (showCalendar == true){
//Show the calendar.
document.getElementById("opencloseimg").src = "images/mins.gif";
//The page we are loading.
var serverPage = "calendar.php";
//Set the open close tracker variable.
showCalendar = false;var obj = document.getElementById(objID);
xmlhttp.open("GET", serverPage);
xmlhttp.onreadystatechange = function() {if (xmlhttp.readyState == 4 && xmlhttp.status == 200) {
obj.innerHTML = xmlhttp.responseText;}
}
xmlhttp.send(null);} else {
//Hide the calendar.
document.getElementById("opencloseimg").src = "images/plus.gif";
showCalendar = true;document.getElementById(objID).innerHTML = "";
}}
account is the JavaScript contained within the functions.js file. A function called >showHideCalendar is created, which will either show or hide the calendar module based upon the
condition of the showCalendar variable. If the showCalendar variable is set to true, an Ajax call to the server is made to fetch the calendar.php script. The results from said script are then
displayed within the calendar page element. You could obviously modify this to load into whatever element you prefer. The script also changes the state of your plus-and-minus image to show true open-and-close functionality.
server-side functionality to create a calendar of the current month. Consider
the following code:
<?php
//calendar.php
//Check if the month and year values exist
if ((!$_GET['month']) && (!$_GET['year'])) {
$month = date ("n");
$year = date ("Y");} else {
$month = $_GET['month'];
$year = $_GET['year'];} //Calculate the viewed month
$timestamp = mktime (0, 0, 0, $month, 1, $year);
$monthname = date("F", $timestamp);
//Now let's create the table with the proper month
?>
<table style="width: 105px; border-collapse: collapse;" border="1"➥
cellpadding="3" cellspacing="0" bordercolor="#000000">
<tr style="background: #FFBC37;">
<td colspan="7" style="text-align: center;" onmouseover=➥ "this.style.background='#FECE6E'" onmouseout="this.style.background='#FFBC37'"> <span style="font-weight: bold;"><?php echo $monthname➥
. " " . $year; ?></span>
</td>
</tr>
<tr style="background: #FFBC37;">
<td style="text-align: center; width: 15px;" onmouseover=➥ "this.style.background='#FECE6E'" onmouseout="this.style.background='#FFBC37'">
<span style="font-weight: bold;">Su</span>
</td>
<td style="text-align: center; width: 15px;" onmouseover=➥ "this.style.background='#FECE6E'" onmouseout="this.style.background='#FFBC37'">
<span style="font-weight: bold;">M</span>
</td>
<td style="text-align: center; width: 15px;" onmouseover=➥ "this.style.background='#FECE6E'" onmouseout="this.style.background='#FFBC37'">
<span style="font-weight: bold;">Tu</span>
</td>
<td style="text-align: center; width: 15px;" onmouseover=➥ "this.style.background='#FECE6E'" onmouseout="this.style.background='#FFBC37'">
<span style="font-weight: bold;">W</span>
</td>
<td style="text-align: center; width: 15px;" onmouseover=➥ "this.style.background='#FECE6E'" onmouseout="this.style.background='#FFBC37'">
<span style="font-weight: bold;">Th</span>
</td>
<td style="text-align: center; width: 15px;" onmouseover=➥ "this.style.background='#FECE6E'" onmouseout="this.style.background='#FFBC37'">
<span style="font-weight: bold;">F</span>
</td>
<td style="text-align: center; width: 15px;" onmouseover=➥ "this.style.background='#FECE6E'" onmouseout="this.style.background='#FFBC37'">
<span style="font-weight: bold;">Sa</span>
</td>
</tr>
<?php
$monthstart = date("w", $timestamp);
$lastday = date("d", mktime (0, 0, 0, $month + 1, 0, $year));
$startdate = -$monthstart;
//Figure out how many rows we need.
$numrows = ceil (((date("t",mktime (0, 0, 0, $month + 1, 0, $year))➥+ $monthstart) / 7));
//Let's make an appropriate number of rows...
for ($k = 1; $k <= $numrows; $k++){
?><tr><?php
//Use 7 columns (for 7 days)...
for ($i = 0; $i < 7; $i++){
$startdate++;
if (($startdate <= 0) || ($startdate > $lastday)){
//If we have a blank day in the calendar.
?><td style="background: #FFFFFF;"> </td><?php
} else {
if ($startdate == date("j") && $month == date("n") &&➥
$year == date("Y")){
?><td style="text-align: center; background: #FFBC37;" ➥
onmouseover="this.style.background='#FECE6E'"➥
onmouseout="this.style.background='#FFBC37'">➥ <?php echo date ("j"); ?></td><?php } else {
?><td style="text-align: center; background: #A2BAFA;" ➥
onmouseover="this.style.background='#CAD7F9'"➥
onmouseout="this.style.background='#A2BAFA'">➥ <?php echo $startdate; ?></td><?php }
}
}
?></tr><?php
}
?>
</table>
The code is set up to allow for alternative years and months, which can be
passed in with the $_GET super-global; but for now,
you are going to concentrate only on the current month. As you progress with the
examples in this chapter, you will see how you can use Ajax to really improve
the functionality of this module and create some very cool applications.
The code itself is fairly simple to decipher. It simply uses the date function in PHP to determine the beginning and end
dates, and then build the calendar accordingly. This is a prime example of using
PHP??s server-side scripting in conjunction with Ajax to create a nice little
application (as shown in Figure 3-1). Next, you??ll work on progressing your
application.
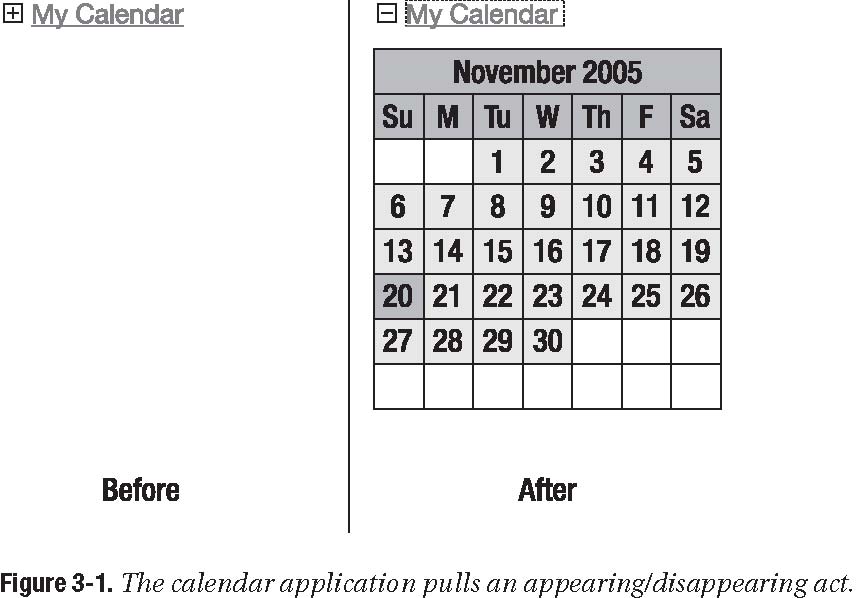
Professional with permission from Apress.