by Marc Plotz
PHP is a powerful tool that allows for processing that may look complex but actually is very simple to do. This article explains a simply way to use Ajax on the client side with PHP on the server side. If you know PHPand a little JavaScriptthis will come very easily to you. If you are not very experienced with JavaScript, don’t worry too much as long as you understand PHP.
Ajax (Asynchronous JavaScript and XML) simply uses a JavaScript method called an
HTTP REQUEST
to communicate with the server without having to refresh the page. When you use Ajax with PHP, PHP does the calculations that it needs to do on the server and sends the result back to the page. You then use JavaScript to display these results in a predefined place.The demo application you will learn how to build in this article is a very simple Suggestion box, just like the one that Google made famous and thus ushered in the Ajax era. It is very simple, and you can download and run (on a PHP server) a working example to see how it really works.
If you do not really understand what is happening on the JavaScript side, don’t feel too badly — JavaScript is not always my friend either!
The Main Page
Let’s start by building the Main Page. This would typically be the web page that requires some form of Ajax functionality. We will call it
index.php
, and it will look like Figure 1.And the result rendered in a browser looks like Figure 2.
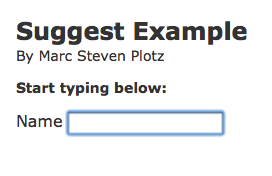
Figure 2. Rendered Result of index.php
If you look at the code represented in Figure 2, you see the following:
Line 1 – Doctype Declaration: Here I just used the new HTML 5 doctype.
Line 5 – JavaScript Include: Include the JavaScript file that is going to do the Ajax HTTP Request.
Line 6 – CSS Block: I’m not too worried about style, so I’ll just chuck in a few little style lines here.
Line 18 – Form: This is the form that will be submitting something at some time or another.
Line 20 – Text Input: Note that this input has a JavaScript function attached to it, which is called every time the user lifts his finger off a previously pressed key.
Line 21 – Hint Div: This is the
Line 5 – JavaScript Include: Include the JavaScript file that is going to do the Ajax HTTP Request.
Line 6 – CSS Block: I’m not too worried about style, so I’ll just chuck in a few little style lines here.
Line 18 – Form: This is the form that will be submitting something at some time or another.
Line 20 – Text Input: Note that this input has a JavaScript function attached to it, which is called every time the user lifts his finger off a previously pressed key.
Line 21 – Hint Div: This is the
div
that will display the hints as they are generated. In effect, the actual content of the ajax.php
page is spit out here.So, as you see in Figure 3, we are calling the JavaScript function in
suggest.js
.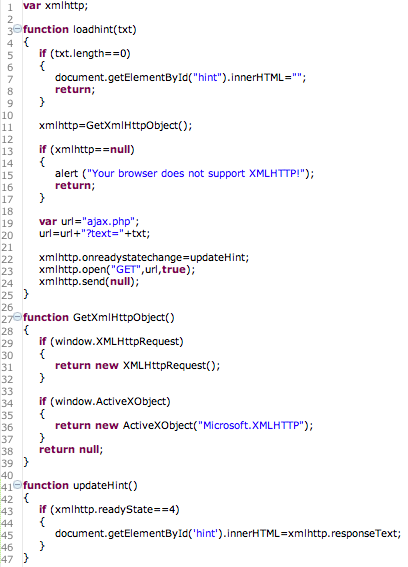
Figure 3. The JavaScript Function in the
suggest.js
If you look at the code represented in Figure 3, you can see the following:
Line 1 – Declare the
Line 3 – Function
Line 19 – Start building the URL so that it looks like
Line 22 – When the state is ready for change, use the
Line 23 – Open the request and set it to use the
Line 24 – Perform the request and relay the result back to the page.
xmlhttp
variable.Line 3 – Function
loadhint
: This function is called every time a user types into the text field. We pass the value of that text field into the function and call it txt
.Line 19 – Start building the URL so that it looks like
ajax.php?text=allan
, where the text
part is what the user has typed so far.Line 22 – When the state is ready for change, use the
updateHint
function to set the new value of the content inside the hint div
. Line 23 – Open the request and set it to use the
GET
method.Line 24 – Perform the request and relay the result back to the page.
To me, most of this JavaScript stuff is really arbitrary and can be used over and over to set up your requests. The more important part, I believe, is the
ajax.php
file (see Figure 4).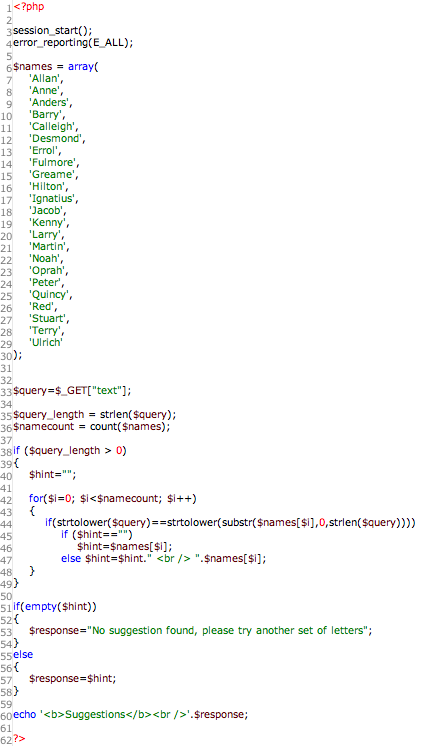
Figure 4. The
ajax.php
FileThis is where the actual processing takes place, and in my mind, this is the important part. You will reuse the JavaScript part over and over again, but the processing page will be different every time.
There are a few things you need to know about this type of Ajax page though. Firstly, remember that the Ajax page is loading after the main page has loaded. Session Variables created in the Ajax page will work only within the confines of the Ajax page. Next, this page should not have a doctype or a head tag, body tag, etc. The HTML rendered inside the Ajax page will never be visible as source when the user
views the main page source.
views the main page source.
Let’s take a closer look at the processing of Figure 4:
Line 6 – Set up an array of names to check against as the user types. This array could come from a database or wherever — it does not really matter.
Line 33 – Get the
Lines 3849 – This is some simple logic to check if what the user has been typing matches anything that is in the
Line 60 – Print out the result of the calculation (this will actually be printed out below the text field that is being entered (see Figure 5).
Line 33 – Get the
$_GET['text']
variable from the URL that has been passed through by Ajax.Lines 3849 – This is some simple logic to check if what the user has been typing matches anything that is in the
$names
array.Line 60 – Print out the result of the calculation (this will actually be printed out below the text field that is being entered (see Figure 5).
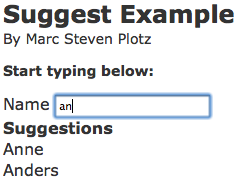
Figure 5. Printout of the Calculation Result
In Conclusion
The whole Ajax interaction deals with loading the content of another page inside the current page using some JavaScript and server-side calculations without the need to reload the page. The JavaScript rarely changes; I have been using that same function for years. Sure, you do change the information being passed into it, and you change the URL being passed to, but that is about it. The
important part is that you know what you are doing with the server-side work so that you get the correct result. The rest, as they say, is just semantics.
important part is that you know what you are doing with the server-side work so that you get the correct result. The rest, as they say, is just semantics.
Until next time,
Download: PHPAjax_SuggestionBox.zip